Installation instructions
[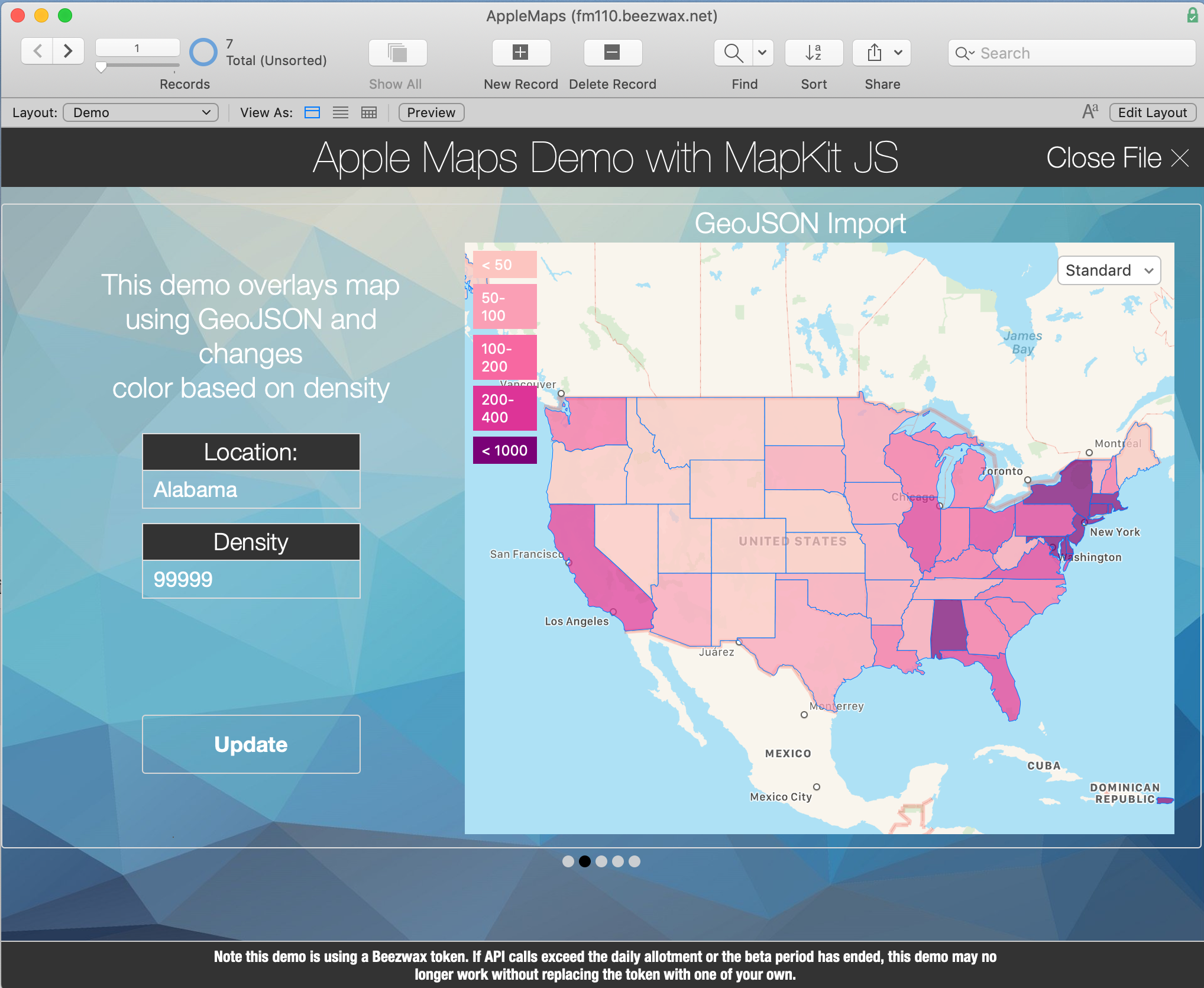](https://blog.beezwax.net/wp-content/uploads/2020/05/Apple-Maps2020.png) ## Account Setup If you do not already have an [Apple Developer account](https://developer.apple.com/account), you must first create one before proceeding. Once you have an account, you must complete these steps before working with maps themselves. 1. Admin access. Make sure you have admin access or you might not be able to see all buttons. Otherwise, ask your admin to obtain: Team Id, Maps ID, Maps Private Key. 2. Team ID. You can find your [Team ID Here](https://developer.apple.com/account/#/membership/) You will need it later. 3. Create a Maps ID. 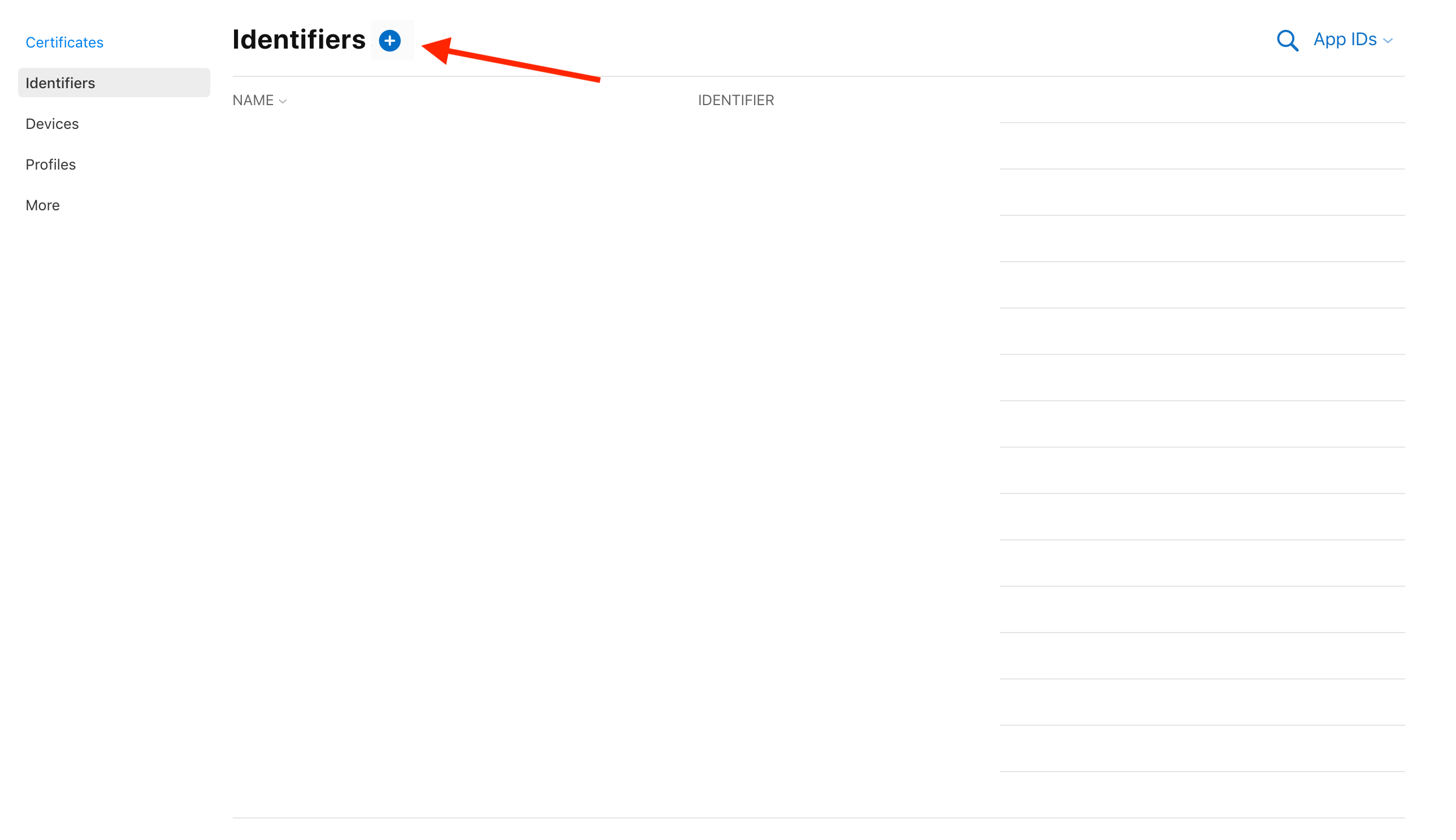 Go to ["Certificates, IDs & Profiles"](https://developer.apple.com/account/resources/identifiers/list). Select Identifiers and click "+" button and fill out the form. For organization, reference, and billing, you may want a different ID for each client or solution. For the identifier, Apple recommends reverse-DNS–style naming, as in _maps.net.beezwax_. 4. Obtain a Maps Private Key. Using your ADP account, go to [all keys page](https://developer.apple.com/account/ios/authkey) and click "+" button and fill out the form. Select checkbox to enable MapKit JS service.  Click configure and assign it to Maps ID you just created.  Go to [all keys page](https://developer.apple.com/account/ios/authkey) again and click download button. You will have a file that looks like AuthKey_Key_zzzKeyIDzzz.p8\. You will need it later. Save it somewhee save. You won't be able to download the same file again unless you start from the beginning. [Official documentation](https://developer.apple.com/documentation/mapkitjs/creating_a_maps_identifier_and_a_private_key) might help you with any problems ## Generate a JSON Web Token. Finally, [generate a JSON Web Token (JWT)](https://mapkitjs.rubeng.nl/ ), which you’ll use to authenticate calls to Apple’s API. Here you will enter your saved Team ID, Maps ID and Your Private Key This should output a string representing the JWT. Copy this into the client-side JavaScript that initializes MapKit JS:
mapkit.init({ authorizationCallback: done => { done( "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ.SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c" ); } }); var map = new mapkit.Map("map"); // Renders Maps onto an element with the div ID of 'map' on your HTML page.
Now you can begin using the MapKit JS API. ## Implementation: FileMaker This is the easy part. Create a few fields for address, search string, or whatever you need for your implementation; add a web viewer to a layout; and integrate the JavaScript. 1. For example, the demo file has a field "StartLocation", which we've inserted into the JavaScript code as: "const FileMakerQuery = '" & Substitute ( Apple Maps::StartLocation ; ¶ ; ", " ) & "';" & ¶ & Note the Substitute() function to replace carriage returns, which Apple Maps does not like, with commas, which it prefers. 2. Next, add a web viewer to the layout. Give it an object name so you can reference it in the CSS for dynamic sizing:
" #map {" & ¶ & " width: " & GetLayoutObjectAttribute ( "map" ; "width" ) & "px;" & ¶ & " height: " & GetLayoutObjectAttribute ( "map" ; "height" ) & "px;" & ¶ & " }" & ¶ &
3. We also factor out the token to a global field, which makes it easier to secure and replace when needed.
" mapkit.init({" & ¶ & " authorizationCallback: done => {" & ¶ & " done(" & ¶ & " '" & Apple Maps::Token & "'" & ¶ & " ); & ¶ & " }" & ¶ & " });" & ¶ &
4. Finally, to make long scripts somewhat more readable, we prefix each line with " and suffix with " & ¶ & . A tool like [BBEdit](https://www.barebones.com/products/bbedit/index.html) makes this quick and easy. Also note you'll either need to use single quotes in your HTML and JavaScript, or escape double quotes. These tweaks allow us to see the script in a more-or-less natural format while still being a valid FileMaker calculation. ## Customization The [MapKit JS documentation](https://developer.apple.com/documentation/mapkitjs/mapkit) provides details on the vast array of customization options available when using this library. Here are two examples to get you started: * [Search](https://developer.apple.com/documentation/mapkitjs/mapkit/search/2974019-search) for a query, such as "coffee shops". * [Lookup](https://developer.apple.com/documentation/mapkitjs/mapkit/geocoder/2973884-lookup) an address to get geographic coordinates. Since it released recently, the MapKit JS documentation is limited. There are few examples of how to use constructors, properties, and functions. You should become comfortable using console.log to output objects to your browser console.
var map = new mapkit.Map("map"); console.log(map); var coordinate = new mapkit.Coordinate(37.415, -122.048333); var span = new mapkit.CoordinateSpan(.016, .016); var region = new mapkit.CoordinateRegion(coordinate, span); console.log(region);
## Demo We've built a simple demo file as a proof-of-concept. It is not meant to illustrate the full range of what is possible, but is, we think, a unique way of looking at map data: 1. Given starting and ending points, highlight all locations along the way that fit our search. 2. Given Census information about population overlay each state with density color 3. Change city zoom limits of the map 4. Use drag&drop feature to move start or end location for our search and build route along the way [Download example file (.zip)](https://blog.beezwax.net/wp-content/uploads/2020/05/AppleMaps2020.fmp12.zip) _Note this demo is using a Beezwax token, which you'll want to replace with your own. While beta MapKit JS is largely free, there is a daily API call allotment limit. If API calls exceed the daily allotment or the beta period has ended, this demo may no longer work without replacing the token with one of your own._ ## Known Issues We ran into minor problems while experimenting with this technique. * When opening a window, the web viewer does not load entirely. Our solution was to apply an OnWindowOpen script trigger. Even though the script can be empty, it seems to be enough to cause the web viewer to draw its content. * The web viewer map on iOS sometimes loses the ability to interpret gestures such as scroll, zoom, and rotate. Switching to a different record seems to resolve the issue most of the time, but additional troubleshooting would be necessary for a production solution. * The CDN copy of the library uses a relative URL to display the Apple logo in the lower left corner, which does not work when using the _file:_ protocol— way FileMaker web viewers render local code. Our solution, which we included in the demo file, was to modify and store a local copy of the library that uses an absolute URL. _Note this local copy will not receive any bug fixes or updates Apple pushes to the CDN.